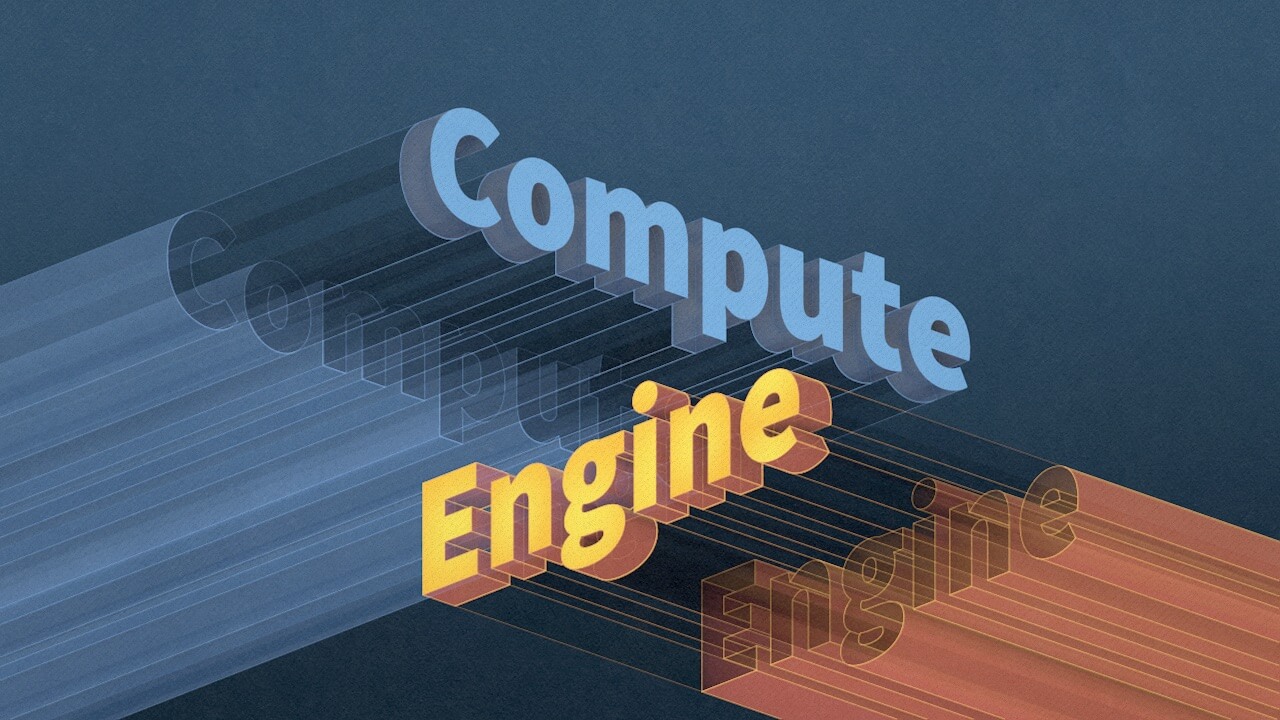
The CortexJS Compute Engine is a JavaScript/TypeScript library for symbolic computing and numeric evaluation of mathematical expressions.
The Compute Engine is for educators, students, scientists and engineers who need to make technical computing apps running in the browser or in server-side JavaScript environments such as Node.
console.log("e^{i\\pi} =", ce.parse("e^{i\\pi}").N().latex);
const expr = ce.parse("(a+b)^2"); console.log(ce.box(["Expand", expr]).evaluate().latex);
const lhs = ce.parse("2x^2 + 3x + 1"); const rhs = ce.parse("1 + 2x + x + 2x^2"); console.log(lhs.latex, lhs.isEqual(rhs) ? "=" : "≠", rhs.latex);
Note: To use the Compute Engine you must write JavaScript code. This guide assumes you are familiar with JavaScript or TypeScript.
The CortexJS Compute Engine manipulates math expressions represented with the MathJSON format.
The Compute Engine can:
- parse and serialize expressions from and to LaTeX
- simplify expressions
- evaluate symbolically expressions
- evaluate numerically expressions
- compile expressions to JavaScript functions
Getting Started
The easiest way to get started is to load the Compute Engine JavaScript module from a CDN.
Using JavaScript Modules
<script type="module">
import { ComputeEngine } from
'https://unpkg.com/@cortex-js/compute-engine?module';
const ce = new ComputeEngine();
console.log(ce.parse("e^{i\\pi}").evaluate().latex);
// ➔ "-1"
</script>
The ESM (module) version is also available in the npm package in dist/compute-engine.min.esm.js
Using Vintage JavaScript
If you are using a vintage environment, or if your toolchain does not support
modern JavaScript features, use the UMD version. You can load the UMD
version by using a <script>
tag.
For example, WebPack 4 does not support the optional chaining operator, using the UMD version will make use of polyfills as necessary.
The UMD version is also available in the npm package in dist/compute-engine.min.js
<script src="//unpkg.com/@cortex-js/compute-engine"></script>
<script>
window.onload = function() {
const ce = new ComputeEngine.ComputeEngine();
console.log(ce.parse("e^{i\\pi}").evaluate().latex);
// ➔ "-1"
}
</script>
Other Versions
A non-minified module which may be useful for debugging is available in
the npm package as dist/compute-engine.esm.js
.
MathJSON Standard Library
The identifiers in a MathJSON expression are defined in libraries. The
MathJSON Standard Library is a collection of functions and symbols that are
available by default to a ComputeEngine
instance.
Topic | |
---|---|
Arithmetic | Add Multiply Power Exp Log ExponentialE ImaginaryUnit … |
Calculus | D Derivative Integrate … |
Collections | List Reverse Filter … |
Complex | Real Conjugate , ComplexRoots … |
Control Structures | If Block Loop … |
Core | Declare , Assign , Error LatexString … |
Domains | Anything Nothing Numbers Integers … |
Functions | Function Apply Return … |
Logic | And Or Not True False Maybe … |
Sets | Union Intersection EmptySet … |
Special Functions | Gamma Factorial … |
Statistics | StandardDeviation Mean Erf … |
Styling | Delimiter Style … |
Trigonometry | Pi Cos Sin Tan … |
In addition to the built-in definitions from the MathJSON Standard Library you can also add your own definitions.
You can also customize the LaTeX syntax, that is how to parse and serialize LaTeX to MathJSON.